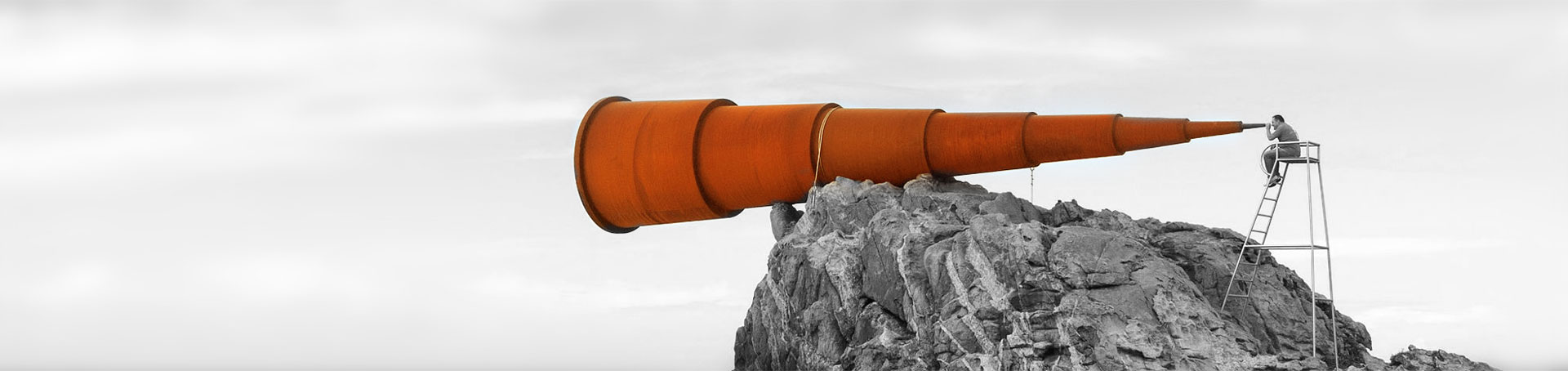
使用SpringBoot框架进行网页应用的开发配置
SpringBoot之web开发
- 概述
- SpringBoot对静态资源(static-location)的映射规则
- 模板引擎
- thymeleaf
- thymeleaf基本概念
- 引入thymeleaf依赖
- thymeleaf使用和语法
- SpringBoot对SpringMVC的web主要的自动配置
- 如何修改SpringBoot的默认配置
- 扩展MVC(不能标注@EnableWebMvc)
- 全面接管SpringMVC(@EnableWebMvc)—不推荐使用
概述
- SpringBoot开发:
1.创建SpringBoot应用,选中需要的场景模块。
2.SpringBoot已经默认将场景模块配置好,只需要在配置文件中指定少量的配置(数据库地址,用户名,密码)就可以运行起来。
3.只需要编写业务逻辑代码。 - 需要掌握自动配置原理:这个场景中SpringBoot默认配置好了什么,能不能修改,能修改哪些配置,能不能扩展。
XxxAutoConfiguration:帮我们给容器中自动配置组件
XxxProperties:配置类,封装配置文件中的内容
SpringBoot对静态资源(static-location)的映射规则
@ConfigurationProperties(
prefix = "spring.resources",
ignoreUnknownFields = false
)
- ResourceProperties可以设置和资源有关的参数,缓存时间等。
/*
* ResourceHandlerRegistry存储用于通过Spring MVC服务静态资源的资源处理程序的注册
* 允许设置为在Web浏览器中高效加载而优化的缓存头
* 可以在Web应用的目录下,类路径等位置之外的位置提供资源
*/
public void addResourceHandlers(ResourceHandlerRegistry registry) {
if (!this.resourceProperties.isAddMappings()) {
logger.debug("Default resource handling disabled");
} else {
Duration cachePeriod = this.resourceProperties.getCache().getPeriod();
CacheControl cacheControl = this.resourceProperties.getCache().getCachecontrol().toHttpCacheControl();
if (!registry.hasMappingForPattern("/webjars/**")) {
this.customizeResourceHandlerRegistration(registry.addResourceHandler(new String[]{"/webjars/**"}).addResourceLocations(new String[]{"classpath:/META-INF/resources/webjars/"}).setCachePeriod(this.getSeconds(cachePeriod)).setCacheControl(cacheControl));
}
String staticPathPattern = this.mvcProperties.getStaticPathPattern();
if (!registry.hasMappingForPattern(staticPathPattern)) {
this.customizeResourceHandlerRegistration(registry.addResourceHandler(new String[]{staticPathPattern}).addResourceLocations(getResourceLocations(this.resourceProperties.getStaticLocations())).setCachePeriod(this.getSeconds(cachePeriod)).setCacheControl(cacheControl));
}
}
}
- 所有/web.jars/**中的资源都在classpath:/META-INF/resources/webjars/中寻找。
- web.jars:以jar包的方式引入静态资源:https://www.webjars.org/
- 访问时,只需要写web.jars下面资源的名称。
- /**:访问当前项目的任何资源(静态资源的文件夹)
classpath:/META-INF/resources/
classpath:/resources/
classpath:/static/
classpath:/public/
/ # 当前项目的根路径
@Bean
public WelcomePageHandlerMapping welcomePageHandlerMapping(ApplicationContext applicationContext) {
return new WelcomePageHandlerMapping(new TemplateAvailabilityProviders(applicationContext), applicationContext, this.getWelcomePage(), this.mvcProperties.getStaticPathPattern());
}
配置欢迎页的映射:
- 欢迎页:静态资源文件夹下的所有index.xml页面,被 /** 映射。
@Configuration
@ConditionalOnProperty(
value = {"spring.mvc.favicon.enabled"},
matchIfMissing = true
)
/*
* ResourceLoaderAware是一个标记接口
* 用于通过ApplicationContext上下文注入ResourceLoader
* 有setResourceLoader()方法
*/
public static class FaviconConfiguration implements ResourceLoaderAware {
private final ResourceProperties resourceProperties;
/*
* ResourceLoader用于返回Resource对象和ClassLoader对象
* - getResource(String location)方法根据提供的location参数返回相应的Resource对象
* - getClassLoader()方法则返回加载这些Resource的ClassLoader
*/
private ResourceLoader resourceLoader;
public FaviconConfiguration(ResourceProperties resourceProperties) {
this.resourceProperties = resourceProperties;
}
public void setResourceLoader(ResourceLoader resourceLoader) {
this.resourceLoader = resourceLoader;
}
/*
* SimpleUrlHandlerMapping是SpringMVC中适应性最强的Handler Mapping类
* 允许明确指定URL模式和Handler的映射关系.有两种声明方式:
* - prop:
* - key: URL模式
* — value: Handler的ID或者名字
* - value:
* - 等号左边是URL模式
* - 等号右边是HandlerID或者名字
*/
@Bean
public SimpleUrlHandlerMapping faviconHandlerMapping() {
SimpleUrlHandlerMapping mapping = new SimpleUrlHandlerMapping();
mapping.setOrder(-2147483647);
mapping.setUrlMap(Collections.singletonMap("**/favicon.ico", this.faviconRequestHandler()));
return mapping;
}
配置喜欢的图标(标签的图标):
- 标签图标:所有的 **/favicon.ico 都是在静态文件夹资源下。
模板引擎
- jsp,velocity,freemarker,thymeleaf
优点 | 缺点 | |
jsp | 1. 功能强大,可以写Java代码 | 1. jsp没有明显的缺点 |
velocity | 1. 不编写Java代码,实现严格的MVC分离 | 1. 不是官方标准 |
freemarker | 1. 不编写Java代码,实现严格的MVC分离 | 1.不是官方标准 |
thymeleaf | 1. 静态html嵌入标签属性,浏览器可以直接打开模板文件,便于后端联调 | 1.模板必须符合xml规范 |
/div>
ul>
/ul>thymeleafthymeleaf基本概念
ul>
/ul>引入thymeleaf依赖
ul>
/ul>
pre>
3.0.2.RELEASE
2.1.1
org.springframework.boot
spring-boot-starter-thymeleaf
/pre>thymeleaf使用和语法
pre>
@ConfigurationProperties(
prefix = "spring.thymeleaf"
)
public class ThymeleafProperties {
private static final Charset DEFAULT_ENCODING;
public static final String DEFAULT_PREFIX = "classpath:/templates/";
public static final String DEFAULT_SUFFIX = ".html";
private boolean checkTemplate = true;
private boolean checkTemplateLocation = true;
private String prefix = "classpath:/templates/";
private String suffix = ".html";
private String mode = "HTML";
private Charset encoding;
/pre>
ul>
1.导入thymeleaf的名称空间
/ul>
pre>
/pre>
p>2.使用thymeleaf语法:
/p>
ul>
/ul>
div>
thymeleaf | jsp | |
片段包含 | th:insert | include |
遍历 | th:each | c:forEach |
条件判断 | th:if | c:if |
声明变量 | th:object | c:set |
任意属性修改 | th:attr | |
修改指定属性默认值 | th:value | |
修改标签体文本内容 | th:text(转义) | |
声明片段 | th:fragment | |
移除声明片段 | th:remove |
/div>
li>
表达式:
/li>
code>Simple expressions: (表达式语法) Variable Expressions: ${...} (获取变量值-OGNL) 1.获取对象的属性,调用方法 2.使用内置的基本对象: #ctx : the context object. #vars: the context variables. #locale : the context locale. #request : (only in Web Contexts) the HttpServletRequest object. #response : (only in Web Contexts) the HttpServletResponse object. #session : (only in Web Contexts) the HttpSession object. #servletContext : (only in Web Contexts) the ServletContext object. 3.内置的工具对象: #execInfo : information about the template being processed. #messages : methods for obtaining externalized messages inside variables expressions, in the same way as they would be obtained using #{…} syntax. #uris : methods for escaping parts of URLs/URIs #conversions : methods for executing the configured conversion service (if any). #dates : methods for java.util.Date objects: formatting, component extraction, etc. #calendars : analogous to #dates , but for java.util.Calendar objects. #numbers : methods for formatting numeric objects. #strings : methods for String objects: contains, startsWith, prepending/appending, etc. #objects : methods for objects in general. #bools : methods for boolean evaluation. #arrays : methods for arrays. #lists : methods for lists. #sets : methods for sets. #maps : methods for maps. #aggregates : methods for creating aggregates on arrays or collections. #ids : methods for dealing with id attributes that might be repeated (for example, as a result of an iteration). Selection Variable Expressions: *{...} (选择表达式,和${}在用法上大体一致) 补充:配合th:object="${session.user}"
Name: Sebastian.
Surname: Pepper.
Nationality: Saturn.
Name: Sebastian.
Surname: Pepper.
Nationality: Saturn.
/code>
li>SpringBoot默认自动配置了SpringMVC
/li>
li>自动配置了
ViewResolver-ContentNegotiatingViewResolver,BeanNameViewResolver(视图解析器:根据方法的返回值得到视图对象,视图对象决定转发、重定向)
1.
ContentNegotiatingViewResolver:组合所有的视图解析器
1.1:如何定制配置-在容器中添加一个定制的视图解析器,ContentNegotiatingViewResolver会自动将定制的视图解析器组合进来
/li>
li>静态资源文件夹路径和
web.jars/li>
li>静态首页访问
/li>
li>favicon.ico
/li>
li>自动注册Converter,GenericConverter,Formatter
1.
Converter:转换器,类型转换使用
2.
GenericConverter:通用转换器,多个源类型和目标类型之间进行转换。
3.
Formatter:格式化器-可以自己定制格式化转换器放在容器中即可以配置
/li>
li>
HttpMessageConverter:SpringMVC用来转换Http请求和响应的。从容器中确定HttpMessageConverters值。可以自己将定制配置的HttpMessageConverter放在容器中即可配置。
/li>
li>
MessageCodeResolver:定义错误代码生成规则
/li>
li>
ConfigurableWebBindingInitializer:初始化web数据绑定器,绑定器把请求数据绑定.可以配置ConfigurableWebBindingInitializer添加到容器中替换默认的
/li>
li>
SpringBoot在自动配置很多组件时,先看容器中有没有已经配置
(@Bean,@Component)好的组件,如果有,就用已经配置好的,如果没有,才自动配置;如果组件可以有多个,将已经配置的和默认配置的组合起来。
/li>
li>编写一个配置类(@Configuration),继承WebMvcConfigurationSupport,不能标注@EnableWebMvc。既保留了所有的自动配置,也可以使用扩展的配置。在做配置时,会导入
/li>
code>@Import({WebMvcAutoConfiguration.EnableWebMvcConfiguration.class})
/code>
strong>EnableWebMvcConfiguration
/strong>:
code>@Configuration public static class EnableWebMvcConfiguration extends DelegatingWebMvcConfiguration {
/code>
strong>DelegatingWebMvcConfiguration
/strong>:
code>//
@Autowired(
required = false
)
public void setConfigurers(List
/code>
strong>参考实现
/strong>:
code>//将所有的WebMvcConfigurer相关的配置都调用一遍 public void addViewControllers(ViewControllerRegistry registry) { Iterator var2 = this.delegates.iterator(); while(var2.hasNext()) { WebMvcConfigurer delegate = (WebMvcConfigurer)var2.next(); delegate.addViewControllers(registry); } }
/code>
li>
容器中所有的WebMvcConfigurer都会起作用。配置的配置类也会被调用。这样Spring的自动配置和扩展配置都会起作用。
/li>
li>禁用SpringBoot对SpringMVC的自动配置,全面对SpringMVC进行配置。在配置类中标注@EnableWebMvc。所有的SpringMVC的默认配置都被禁用了。
/li>
li>
@EnableWebMvc:
/li>
code>@Import({DelegatingWebMvcConfiguration.class}) public @interface EnableWebMvc { }
/code>
code>@Configuration public class DelegatingWebMvcConfiguration extends WebMvcConfigurationSupport {
/code>
code>@Configuration @ConditionalOnWebApplication( type = Type.SERVLET ) @ConditionalOnClass({Servlet.class, DispatcherServlet.class, WebMvcConfigurer.class}) @ConditionalOnMissingBean({WebMvcConfigurationSupport.class}) //当容器中没有此组件时,此自动配置类才生效 @AutoConfigureOrder(-2147483638) @AutoConfigureAfter({DispatcherServletAutoConfiguration.class, TaskExecutionAutoConfiguration.class, ValidationAutoConfiguration.class})
/code>
li>@EnableWebMvc将WebMvcAutoConfigurationSupport导入进来,不包括SpringMVC的功能。
总结:
/li>
li>
多多学习SpringBoot中的XxxConfigurer,进行扩展配置/li>